CSCE 120
JavaScript
This section will cover the following
-
Introduction to JavaScript
- Introduction to JavaScript
- Introduction to Development Tools
- Semantics, Syntax and Types
- Numbers, Expressions and Operators
- String and Dates
- Program & Control Flow
- Loops and Iteration
- Functions
- Arrays and Objects
- Working with Collections
- Promises and Error Handling
- Introduction to Design Patterns
- Modules
-
Web Programming with JavaScript
- BOM and DOM
- JavaScript Events
- Forms
- Data Access Using HTTP
- JavaScript APIs
- Introduction to JavaScript Security
- Introduction to JavaScript Libraries and Frameworks
Introduction to JavaScript
Developed by Netscape in mig 1990s for adding dynamic elements to HTML.
Originally knwn as "LiveScript"; later changed to JavaScript.
JavaScript Versions: Both ECMAScript 5 (ES5) and ECMAScript 6 (ES6) versions are widely used. Some features of ES6 are not supported by all browsers.
JavaScript is a high-level and interpreted programming language.
High-level means it has all the funtionalities of well received language. It has high abtraction from the users which makes it easy to use.
In contrast, C prgramming language is a low-level language which is more complicated but gives you more control over low-level processing like memory management.
Interpreted means it does not need to be compiled before it's executed, it is interpereted at runtime.
JavaScript is NOT Java
JavaScript is a cross-platform scripting language
JavaScript is object-oriented
JavaScript is used to make webpages interactive such as animations, clickable / touchenable elements and more
One of the most popular programming language in the world
JavaScript is used to develop these type of applications
- Web
- Desktop (using Electron)
- Smartphone and Tablet (Cordova, Ionic, ReactNative, more)
- Server Side Apps (using NodeJS)
- Games
Introduction to Development Tools
Online Tools
Online tools are great for demonstrations, proof of concepts and sharing solutions.
- jsfiddle.net
- CodePen
- Plunker (new version next.plnkr.co)
- More online...
Demos
Development Environment and Tools
There are many editors and tools that help accelerate software development; some tools are specifically targeted for web development. Within the web development, some tools are geared toward front-end development and some toward back-end (server side) development. In this class we will use the latest version of Visual Studio (VS) which is free. VS is designed for full-stack development where both front-end and back-end development can be done. We will also use several tools such as jQuery and Bootstrap when necessary.
Additional tools
Demo
Semantics, Syntax and Types
JavaScript uses the Unicode character set and is case-sensitive. If you are familiar with one of the C based languages, you will recognize JavaScript code easily. According to Mozilla Developer Network (MDN), "JavaScript borrows most of its syntax from Java, C and C++, but is also influenced by Awk, Perl and Python"
Variable Declaration
JavaScript presents three key words for variable declaration: var, let, const
var firstName; // OR var firstName, lastName;
let firstName; // OR let firstName, lastName;
// When using var and let, the variable may be optionally initialized i.e: let firstName = "John";
The var statement declares a variable, while let declares a block scope local variable and was introduced with ES6.
Which one do I use?
Using let is less error prone, however some older browser may not support let because it was introduced with ES6. Developers need to where the JavaScript will run before they use new ES6 features. If possible use let.
Consult
const
const stands for constant and are block-scoped, much like variables defined using the let statement. The value of a constant can't be changed through reassignment, and it can't be redeclared. const variables are read-only.
Demo
variable names must start with a letter, underscore (_), or dollar sign ($); subsequent characters can also be digits (0-9)
JS is case-sensitive
// Three different variable names
var firstName;
var FirstName;
var FürstName; // uses the Unicode character set - ü
Comments in JavaScript
Comments are discarded during script execution
// a one line comment
/* this is a longer,
* multi-line comment
*/
undefined
When a variable is declared with no assigned value specified has the value of undefined
Demo
Variable hoisting
Variable hoisting is an unusual thing about variables in JavaScript. Hoisting make it possible to refer to a variable declared later, without getting an exception. variables declared using var are in a sense "hoisted" or lifted to the top of the function or statement. variables that are hoisted always return a value of undefined, even they are declare and initialize after referencing the variable.
Demo
let and const are hoisted but not initialized. Referencing the variable in the block before the variable declaration results in a ReferenceError not undefined With let and const variables are in a "temporal dead zone" from the start of the block until the declaration is processed.
Function hoisting will be covered in Functions section
Semantics, Syntax and Types
JavaScript categorizes data types in to two
- Primitive Types
- Object
Object is a subject that will be covered later
Primitive Types are as follows:
- Number. An integer or floating point number
- String. A sequence of characters that represent a text value
- Boolean. true and false
- undefined. A top-level property whose value is not defined
- null. A special keyword denoting a null value
- BigInt. An integer with arbitrary precision
- Symbol. A data type whose instances are unique and immutable. Introduced by ES6
Numbers, Expressions and Operators
According to MDN, "In JavaScript, numbers are implemented in double-precision 64-bit binary format IEEE 754 (i.e., a number between ±2−1022 and ±2+1023, or about ±10−308 to ±10+308, with a numeric precision of 53 bits). Integer values up to ±253 − 1 can be represented exactly."
Also, the number type has three symbolic values: +Infinity
, -Infinity
, and NaN
(not-a-number). And Number can also be used as an object (objects will be covered later in this class)
BigInt was introduced by ES6, provides the ability to represent integers that may be very large. BigInt and Number values cannot be mixed and matched in the same operation.
Binary, Octal numbers, Hexadecimal numbers can also be represented in JavaScript.
Demo
JavaScript math operations follow the same order of precedence as algebra. The order in which mathematical operations must be executed: Parentheses, Exponents, Multiplication, Division, Addition, Subtraction.
Operators
JavaScript has several types of operators. Below are the most frequently utilized types of operators
- Assignment operators
- Arithmetic operators
- Comparison operators
- Logical operators
- Conditional (ternary) operator
- String operators
- Unary operators
- Relational operators
Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
let name = "John";
x = y;
Arithmetic operators
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value.
JavaScript uses the following operators for arithmetic;
- Addition
(+)
- Subtraction
(-)
- Multiplication
(*)
- Division
(/)
- Modulus (division remainder)
(%)
- Increment
(++)
- Decrement
(--)
- operands are equal and of the same type. - Unary negation
(-)
- Unary plus
(+)
Arithmetic operators follow the operator precedence, determined in algebra.
Comparison operators
A comparison operator compares its operands and returns a logical value based on whether the comparison is true.
JavaScript uses the following operators for comparison;
- Equal
(==)
- Not Equal
(!=)
- Strict Equal
(===)
- operands are equal and of the same type. - Strict Not Equal
(!==)
- Greater than
(>)
- Greater than or equal
(>=)
- Less than
(<)
- Less than or equal
(<=)
Logical operators
Logical operators are used to determine the logic between variables or values
JavaScript uses the following logical operators;
- Logical AND
(&&)
- Logical OR
(||)
- Logical NOT
(!)
Conditional (ternary) operator
condition ? val1 : val2
let status = (age >= 18) ? "adult" : "minor";
String operators
console.log("John " + "Smith"); // console logs the string "John Smith".
var name = "John";
name += " Smith";
console.log(name); // console logs the string "John Smith".
Unary operators
Unary operators have only one operand. The most common used unary operator is typeof
Other unary operators are delete and void
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
There are two main relational operators: in and instanceof
Expressions
An expression is a valid unit of code that resolves to a value. JavaScript expressions can be seperated into two categories: Assigned expressions and Evaluated expressions.
Assigned expressions are self explantory. A value is assigned to a variable x = 5
The value of the variable becomes 5.
Evaluated expressions are more involved. These type of expressions use more than one operations and operators to evaluate the result which may or may not be assigned to a variable. In other word, evaluated expressions are code snippets that result in a single value. JavaScropt has several expression categories: Aritmatic, String, Logical, Left-hand-side, and Primary expressions.
We will dig deep inot expressions in functions section.
String and Dates
Strings
JavaScript treats strings as a sequence of characters that represent a text value. A string can be any text inside double or single quotes. Each element in the String occupies a position in the String. The first element is at index 0, the next at index 1, and so on. Any data type can be convertd to string.
Demo
JavaScript treats stringsas objects which gives string methods and properties. Most common used String Properties are
- length : Returns the length of a string
- prototype : Makes it possible to add properties and methods to a string(object)
JavaScript string have a large list of methods. Below are some of the most common use methods
- charAt() --> Returns the character at the specified index (position)
- concat() --> Concatinate two or more string to make new string
- includes() --> Checks whether a string contains the specified string/characters
- indexOf() --> Returns the position of the first found occurrence of a specified value in a string
- replace() --> Searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced
- slice() --> Extracts a part of a string and returns a new string
- split() --> Splits a string into an array of substrings
- substr(), substring() --> Extracts a sub string from a string
- toLocaleLowerCase() --> Converts to lowercase letters, according to the host's locale
- toLocaleUpperCase() --> Converts uppercase letters, according to the host's locale
- toLowerCase() --> Converts a sto lowercase letters
- toUpperCase() --> Converts uppercase letters
- trim() --> Removes whitespace from both ends of a string
- toString() --> Converts to a string(String object)
Dates
JavaScript does not have a Date Type, however Date Object provides functionality to work with dates. Date objects contain a number that represents milliseconds since January 1, 1970 UTC. When used in browsers, JavaScript will use the browser's time zone and display a date as a full text string:
Date Object
Date objects are created with the new Date()
constructor. When an object is created, its default method is called.
Some objects may have more than one default methods. These methods are known as constructor.
Which constructor gets called is based on the constructors' signature.
JavaScript Date object has several construtors:
new Date()
new Date(year, month, day, hours, minutes, seconds, milliseconds)
new Date(milliseconds)
new Date(date string)
Every instances of Date object inherits from Date.prototype. The prototype object of the Date constructor can be modified to affect all Date instances.
There are also several methods provided with the date object. These methods allow date operations and manipulations.
To the full list of properties and methods on Date object, check ou MDN or open your .js file in Visual Studio, create an instance of Date object as seen in the following image.
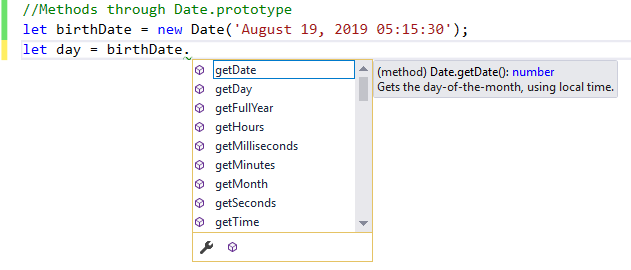
Program & Control Flow
JavaScrip programs run top to bottom; every instruction is executed. However, most programs include statements or blocks of code which alters the execution sequence. In this section we will cover JavaScript statements.
Block statement
Block statements are the most basic and easy to understand. Block are identified by a pair of curly brackets
statement 1;
statement 2;
statement 3;
Another easy to understand statement is used with loops
var nameES5 = "John";
{
var nameES5 = "James";
}
console.log(nameES5); // outputs "James"
let nameES6 = "John";
{
let nameES6 = "James";
}
console.log(nameES6); // outputs "John" -- What? I axpected "James"
Another easy to understand statement is used with loops
//while loop
while (x < 10) {
displayValue();
x++;
}
Important: block scope was introduced to JavaScript via ES6 (ECMAScript2015). In ES5, variables introduced within a block are scoped to the containing function or script, and the effects of setting them persist beyond the block itself. In other words, block statements do not define a scope (Source: MDN)
Conditional statements
The if stetement is a straightforward example
if (person.age > 34) {
eligibleForDiscount();
addToGolfTeam();
}
What's going on: As JavaScript executes instructions top to borrom, it will run into the if statement.
The eligibleForDiscount();
will only be executed when the if condition is true.
if block can inclued one or more statements. if may have one or more statements for the condition being false. This is known as else
condition.
Also, the if statement may include multiple conditions. Each of which may have one or more statements. There may also be nested if.
if (person.age > 34) {
eligibleForDiscount();
addToGolfTeam();
}
else if (person.age > 25 && person.age < 34)
{
if (person.age < 30) {
addToSoccerTrainer(); // will help other player in the team
}
addToSoccerTeam();
}
else {
addToFootballTeam();
}
switch
The switch statement works very similar to the if statement: It a program to evaluate an expression and attempt to match the expression's value to a case label.
switch (expression) {
case label_1:
statements_1
[break;]
case label_2:
statements_2
[break;]
default:
statements_def
[break;]
}